Unity "Blink Pulse" Sprite Animation
Create a simple "blink pulse" sprite animation in Unity with this lightweight script. Adjust minimum alpha, maximum alpha, and cycles per second to control the fade effect. Attach it to your sprite GameObject for an easy, customizable pulsating animation that enhances your game's visuals!
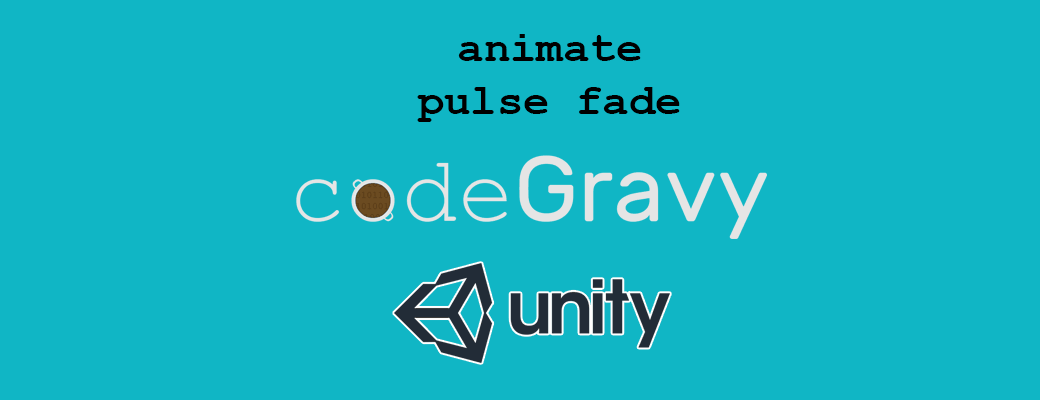
Learn how to create a simple and effective "blink pulse" sprite animation in Unity. This step-by-step guide will teach you how to fade a sprite's alpha in a pulsating pattern using a lightweight script.
Why Use a Blink Pulse Animation?
Adding a blink pulse effect can bring life to your game objects, making them visually dynamic and engaging. Whether you're designing a glowing button or a magical aura, this effect is versatile and enhances the overall user experience.
The Script: Lightweight and Customizable
In my script folder, I keep a "micro" folder filled with tiny, reusable scripts like this one. These scripts are designed for quick implementation—just attach them to your GameObjects and tweak the parameters as needed. Here's the code:
public class blinkPulse : MonoBehaviour {
private SpriteRenderer sr;
public float minimum = 0.3f;
public float maximum = 1f;
public float cyclesPerSecond = 2.0f;
private float a;
private bool increasing = true;
Color color;
void Start() {
sr = gameObject.GetComponent<SpriteRenderer>();
color = sr.color;
a = maximum;
}
void Update() {
float t = Time.deltaTime;
if (a >= maximum) increasing = false;
if (a <= minimum) increasing = true;
a = increasing ? a += t * cyclesPerSecond * 2 : a -= t * cyclesPerSecond;
color.a = a;
sr.color = color;
}
}
Key Parameters
The script has three adjustable parameters:
minimum
: The lowest alpha value (e.g.,0.3
for 30% opacity).maximum
: The highest alpha value (e.g.,1
for full opacity).cyclesPerSecond
: Controls the speed of the pulse; higher values mean faster animation.
How to Use It
- Attach the script to your sprite GameObject in Unity.
- Adjust the
minimum
,maximum
, andcyclesPerSecond
values in the Inspector panel to suit your needs. - Hit play, and watch your sprite come alive with a smooth pulsating glow!
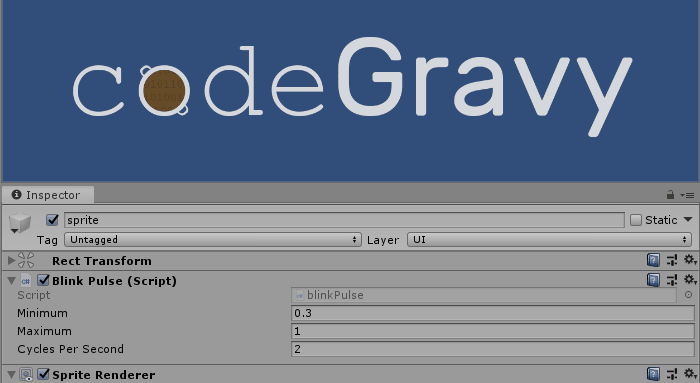
Attach the script to your sprite GameObject, and you are good to go!
More simple to implement, yet immersive effect:
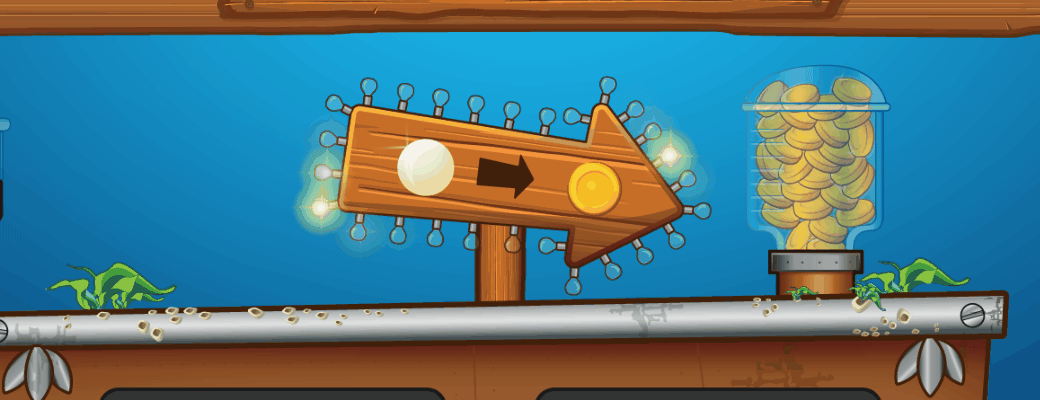
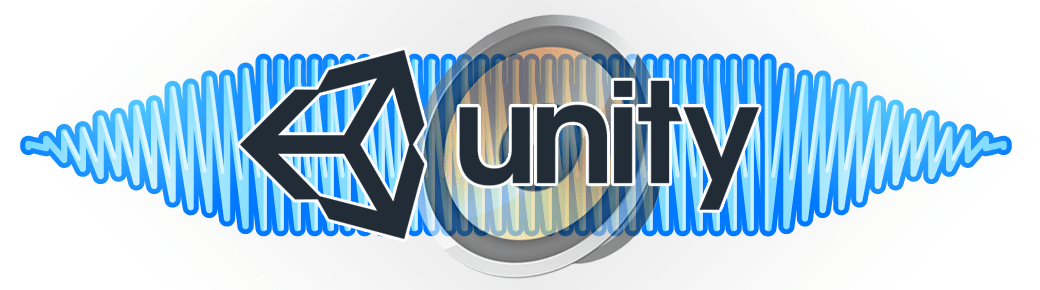