Unity audio fade in/out function (C#)
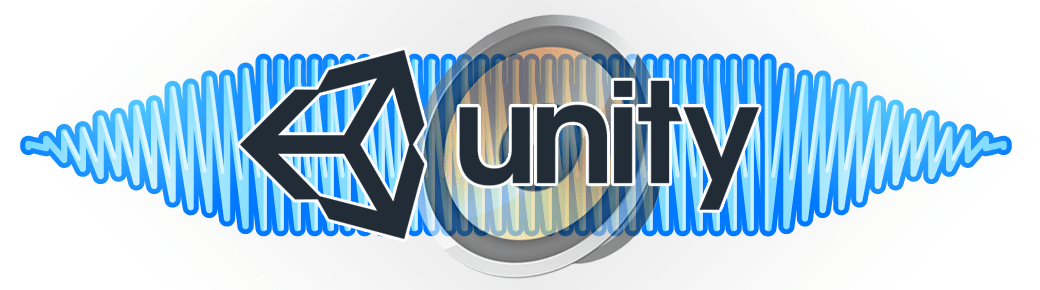
Easiest way to fade sound in your unity project using scripting. Create AudioHelper.cs and paste the code below.
using System.Collections;
using UnityEngine;
public static class AudioHelper {
public static IEnumerator FadeOut(AudioSource audioSource, float FadeTime) {
float startVolume = audioSource.volume;
while (audioSource.volume > 0) {
audioSource.volume -= startVolume * Time.deltaTime / FadeTime;
yield return null;
}
audioSource.Stop();
}
public static IEnumerator FadeIn(AudioSource audioSource, float FadeTime) {
audioSource.Play();
audioSource.volume = 0f;
while (audioSource.volume < 1) {
audioSource.volume += Time.deltaTime / FadeTime;
yield return null;
}
}
}
How to use in your project
Since this function is an IEnumerator it must be started as a coroutine. This example I get the audiosource from the gameobject the script is attached to. I set fadeTime to 2 seconds.
private AudioSource soudtrackAudioSource;
private float fadeTime = 2f;
void Start () {
soudtrackAudioSource = GetComponent<AudioSource>();
}
StartCoroutine(AudioHelper.FadeIn(soudtrackAudioSource, fadeTime));
StartCoroutine(AudioHelper.FadeOut(soudtrackAudioSource, fadeTime));